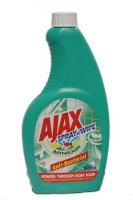
JQuery is a great JavaScript framework that makes web developer life much easier. But like all framework, you need to learn its gotchas in order to work effectively with it. Here is one of those gotchas –
Jquery POST method lets you create Ajax HTTP POST request to the server. It is actually a shorthand to the JQuery Ajax method:
$.ajax({
type: "POST",url: "save.php",
data: "param1="+paramValue1
+"¶m2=paramValue2",
complete: function(){ }, //manage the complete if needed
success: function(){}}//get some data back to the screen if needed
});
The problem
When executing the AJAX call, only part of the data is passed to the server and the rest vanishes. You usually see that some or all of the parameters you tried to pass are missing or cut in the middle.
The cause
JQuery uses ‘&’ as a separator between the parameters. If you have a ‘&’ within your key or value parameters, then the JQuery AJAX request gets really messed up.
The solution
Encode the parameters, replace & with %26 which is the standard encoding for that character.
Semi-Automatic
Use .replace(/&/g, “%26”) –
Here is a working example:
$.ajax({
type: "POST",url: "save.php",
data: "param1="+paramValue1.replace(/&/g, "%26")
+"¶m2=paramValue2.replace(/&/g, "%26")",
complete: function(){ }, //manage the complete if needed
success: function(){}}//get some data back to the screen if needed
});
Fully Automatic
A more elegant way is to slightly change the way we call the meethod and let JQuery do that encoding for you –
Here is a working example:
$.ajax({
type: "POST",url: "save.php",
data: { "param1": paramValue1,
"param2": paramValue2 },
complete: function(){ }, //manage the complete if needed
success: function(){}//get some data back to the screen if needed
});